Programming
The importance of programming
Computer programming is a skill that everyone can, and should, learn. Programs are useful for automating tasks, analyzing data, and mocking up and prototyping devices, and also for helping us break down and debug problems. The ability to think like a programmer is just one more tool in your box!
Progamming languages
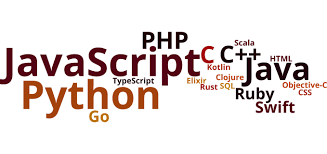
There are many programming languages, each with important pros and cons. Compiled languages, like C++, run fast code, but take the longest to learn how to write. Statically interpreted languages, like Java, are cross-platform, are easier to write, but don't run as fast. Dynamically interpreted languages, like Python and Matlab, are the fastest to write, but run the slowest.
How programs are written
The process of writing a program starts with the development environment, or IDE. Popular IDEs are Visual Studio, Xcode, Netbeans, and Eclipse. Nice IDEs have helpful features like autocomplete.
Version Control
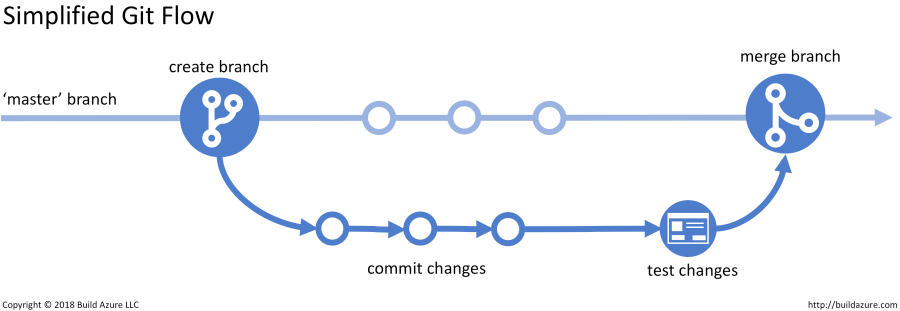
Most programs are written in collaboration. A lot of effort is put into making sure programmers can understand each other's code, and making sure big projects written by many people can be spliced together. Version control software, like git, is a standard way to identify who is working on what, merging changes, and identifying and fixing bugs.
Python
We will use Python to explore some introduction to programming. Python is a popular dynamic language (if you are familir with Matlab you'll see a lot of similarities). Python code is interpreted as it runs, so it is not a fast language, but it is easy to do lots of interesting things in Python with very little experience.
Python in the browser
One way to learn Python is to run it directly in your browser, for instance on trinket.io. Every week there are more websites like this that have tutorials and code as you go lessons, with nothing to download!
Python on your computer
The most reliable way to run Python is by installing it on your computer. Python itself is very lightweight, the real power comes from add-on libraries that do statistics, make graphics, and do machine learning. You can download Python and the most popular libraries using Anaconda.
Python using Mu
But we'll use something a little simpler, a program called Mu (pronounced moo), from https://codewith.mu/en/. Mu let's you program traditional Python, and also CircuitPython on microcontrollers, and games using Pygame Zero. It installs Python 3 and some common libraries. Mu is an IDE with some basic debugging tools, plenty to get started with.
Open Mu and click the Mode button in the top left. Select Python 3. You can see the text window with a comment, and some buttons, like Run. Python files are saved with the extension .py.
Repl mode
As a dynamic language, you can run Python one line at a time, without even needing to write a .py file. This mode is called REPL, or read-evaluate-print-loop. Click the REPL button and you'll see a line at the bottom that says In [1]:. There you can type lines of Python, one at a time, and see what happens. Try making a variable, like a=4, and pressing enter. Then press a and enter, and Python will tell you the value of a. Pretty neat!
Try doing some math. Do fractions work like decimals or integers? What happens when you divide by 0?
Make an array (in Python called a list) like c=[1,2,3]. What is the value of c[1]? What is the value of c[-1]?
Python built-in functions
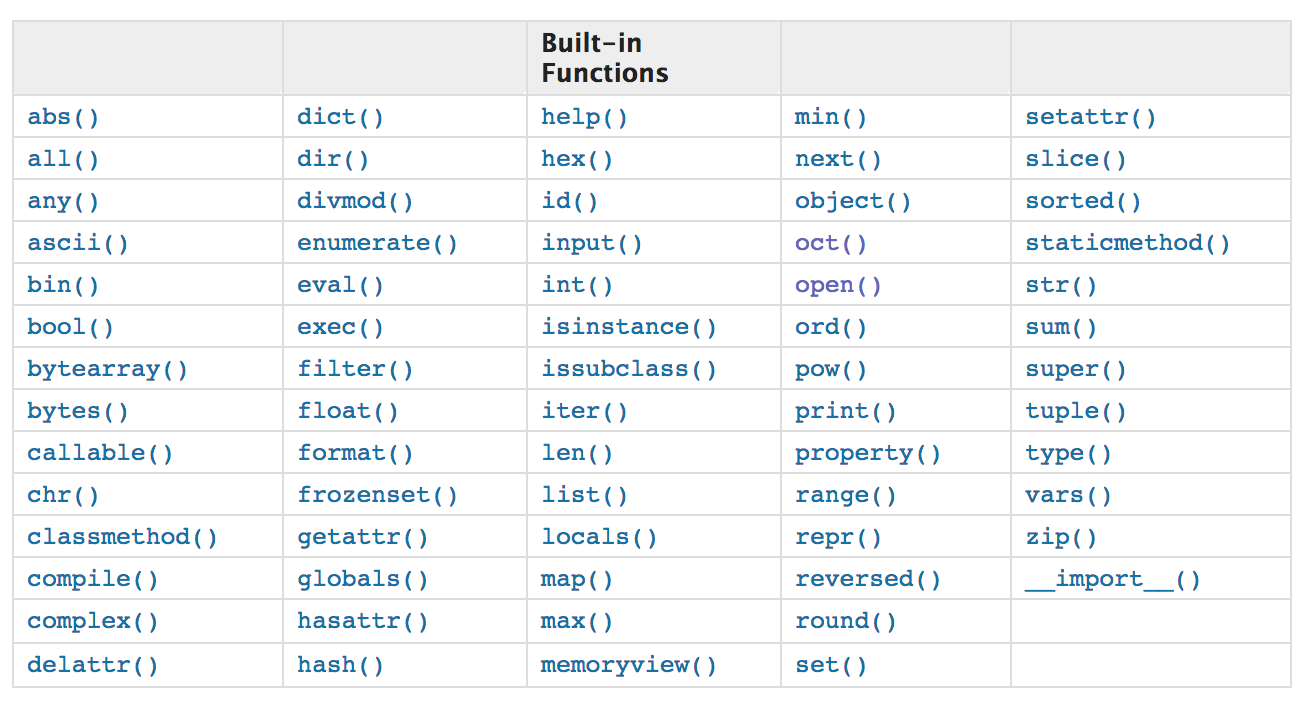
Python has a limited set of built-in functions. Try using print() on a list. Try using sum() on a list. Try making a list with letters and numbers, like k=[1,'t',3]. What happens when you sum(k)? How do you sum 't' and 1?
Python conditional statements
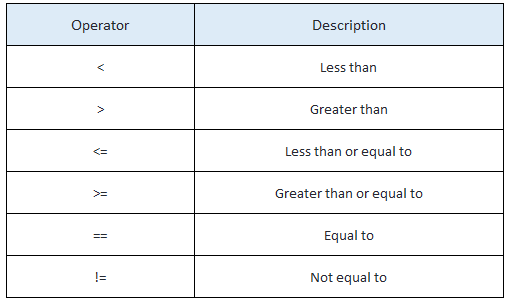
So far it looks like Python is a fancy calculator. As a programming language, it can run special syntax to do comparisons and loops. Try an if() statement. Note that statements like this end in : and the next line needs to be tabbed, and every line that should happen next is also tabbed.
Syntax
Repl mode is great for testing single lines of code, but to be efficient, we should write a program and save it as a .py file, then hit the Run and Stop buttons. Edit the file so that you make a variable and test its value using an if() statement. If the statement is true, print() something.
Edit the program to ask the user for a number, using the intput() function. Immediately turn the value into an integer using int(). If the value is 4, print Yes!
Write a function
If you find yourself using the same code over and over, you should probably turn it into a function. A function is a block of code that takes and input and returns an output. Functions go at the top of your file, using the def to start, and return to end. Try the following code:
def doubleIt(varIn):
return varIn*2
def main():
a = 2
b = doubleIt(a)
print(b)
main()
Python libraries
Libraries of extra functions are what makes Python so powerful. For example, NumPy has mean(), std(), etc. To add the NumPy library, use Import numpy, and then you can use the functions like numpy.mean(). Sometimes we shorted the name of the library to make less typing, so Import numpy as np, and then you can use np.mean().
Try making a list of numbers and taking the mean.
You can see the libraries installed with Mu in a folder that looks like C:\Users(username)\AppData\Local\Mu\pkgs.
Make a plot
The library matplotlib makes plots that look like Matlab plots, using similar syntax. Try:
import matplotlib.pyplot as plt
x = [1,2,3,4,5]
y = [6,7,8,9,0]
plt.plot(x,y,'ro-')
plt.show()
Loops
A while() loop will continue until its condition is met. A for() loop will perform an action a set number of times. Both can be used to iterate over lists or actions.
For example:
a=5
while a>0:
print(a)
a = a - 1
for x in range(5):
print(x)
Use a for() loop to build a list that is the sine(t), then plot the list.
Import data from a file
One way to get data into Python is to save it as a comma separated file type (csv). Then you can loop through every row and analyze it. For example:
import csv
with open('data.csv') as f:
reader = csv.reader(f)
for row in reader:
print(row)
This will print out the entire csv file.
Want to try something cool?
You can get the number of entries in every CTA train stop every day since 2001 from the CTA open data portal clink export->CSV (warning, 32Mb file size). Put the csv file in the same folder as your .py file (usually in a folder called mu_code).
The second element is the name of the station, and the fifth is how many entries there were that day.
Loop through and count how many entires there have been at Noyes since 2001:
total = 0
import csv
with open('cta2020.csv') as f:
reader = csv.reader(f)
for row in reader:
data = row
if (row[1] == 'Noyes'):
total=total + int(row[4])
print(total)
Which station is busier, Foster or Noyes?