Neopixel
We can set the brightness of a single color of LED using the pulseio function. How can we control a full color RGB LED, or a lot of LEDs, without using all the pins of the ItsyBitsy? A Neopixel is a brand of WS2812B RGB LEDs that can be controlled with a single pin, and chained so that a whole strip can be controlled individually for brightness and color, still using just one pin. Neopixels can be purchased individually, on long strands, and in all kinds of arrays of shapes.
Wire the Neopixel
The Neopixel board in the kit takes 5V, ground, and any pin for DIN. The other end of the board has pads to solder connections to the next set of Neopixels, connecting DOUT to the next DIN. I have pre-soldered header pins on so you can plug the board into your breadboard.
Run the example code
The Neopixels need a library called neopixel.mpy and adafruit_pypixelbuf.mpy from the Adafruit library bundle, place them in the lib folder of the CircuitPython drive.
Add the library and object
import neopixel
pixel_pin = board.D5
num_pixels = 8
pixels = neopixel.NeoPixel(pixel_pin, num_pixels, brightness=0.3, auto_write=False)
Some cool color functions:
def wheel(pos):
# Input a value 0 to 255 to get a color value.
# The colours are a transition r - g - b - back to r.
if pos < 0 or pos > 255:
return (0, 0, 0)
if pos < 85:
return (255 - pos * 3, pos * 3, 0)
if pos < 170:
pos -= 85
return (0, 255 - pos * 3, pos * 3)
pos -= 170
return (pos * 3, 0, 255 - pos * 3)
def rainbow_cycle(wait):
for j in range(255):
for i in range(num_pixels):
rc_index = (i * 256 // num_pixels) + j
pixels[i] = wheel(rc_index & 255)
pixels.show()
time.sleep(wait)
And call the function:
rainbow_cycle(0) # Increase the number to slow down the rainbow
The Neopixels can be harshly bright, so I like to put something over them to diffuse the color, like a sheet of white paper, tissue, or cotton.
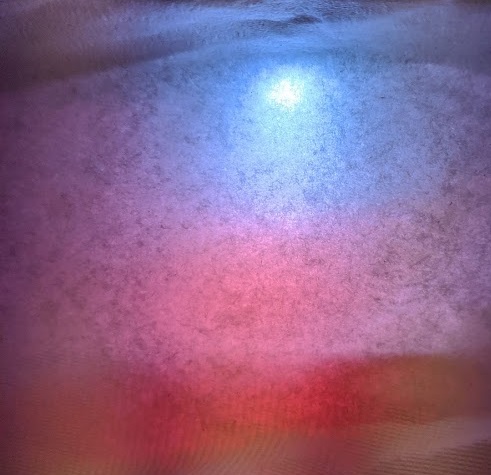